Context
Building microservice in front-end is getting popular, there is even a book about it.
If you are building a front-end platform for public or internally for your company, most likely you will encounter the need to enable multiple teams to develop and release in parallel. To ensure productivity, your platform should provide solution for isolated team code base as well as release pipeline.
Which means the final product that present to end users will be a master website loads a bunch of other websites into its DOM. In order to provide enough security and avoid one sub app crash the entire website, you will have to use iframe as the container, as Web Component is far from mature.
One of the major issue with iframe is, communicate over the boundary is a huge pain. As “postMessage” API is like UDP, it is very hard and cumbersome even just exchange some simple text.
“Bridge” for the rescue
I created an open source package Bridge, to provide developer friendly protocols for developer to easily communicate across boundary.
Expose an function/API
When you want to expose a function/API for others to invoke, similar to a backend API, with Bridge we just have to create a resolver and have your API as a function inside it.
// Here is a sample resolver that expose an "echo" API
class HostSampleResolver implements IResolver {
public name: string = "HostSampleResolver";
public echo(inputs: { message: string }, from: string): Promise<any> {
return new Promise((resolver) => {
setTimeout(() => {
resolver({ data: `echo from host: ${inputs.message}` });
}, 500);
});
}
}
To invoke above API, all your need is one function call. Name the resolver we want to invoke, the API we want to call, and pass in an input if there is any.
const response = await client.invokeResolver<string>("HostSampleResolver", "echo", { message: "message from client" });
Subscribe to an event
Bridge also supports pub-sub. Simply subscribe to an event that the other side might push over any notification.
client.subscribe("host-event", (inputs: any) => {
console.log("Client - sub ===>", inputs);
});
To push notification to subscriber is just one function away
host.broadcastEvent(`host-event`, "YOLO");
Get your hand dirty
You can look at the full and runnable sample code from Bridge’s repository. To run it, please follow instructions from README.md
Here is a preview on what you will expect from our sample, where we have a website loads another website into a DIV as well as an IFRAME, and both sub-apps from the DIV and the IFRAME are communicating with the master website via Bridge.
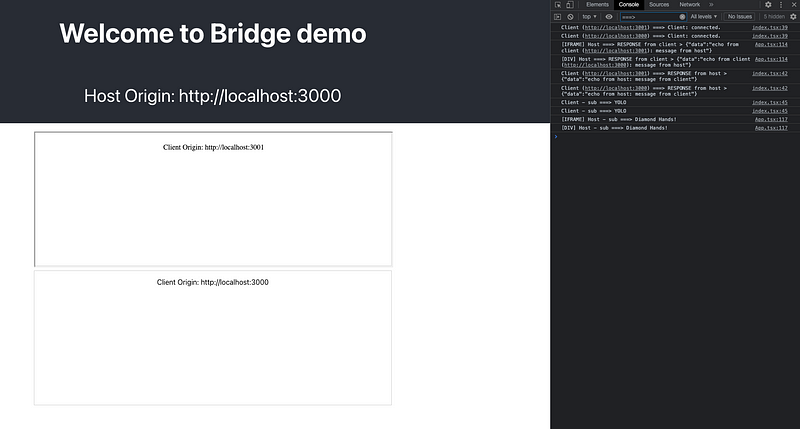